Sep 18 2023
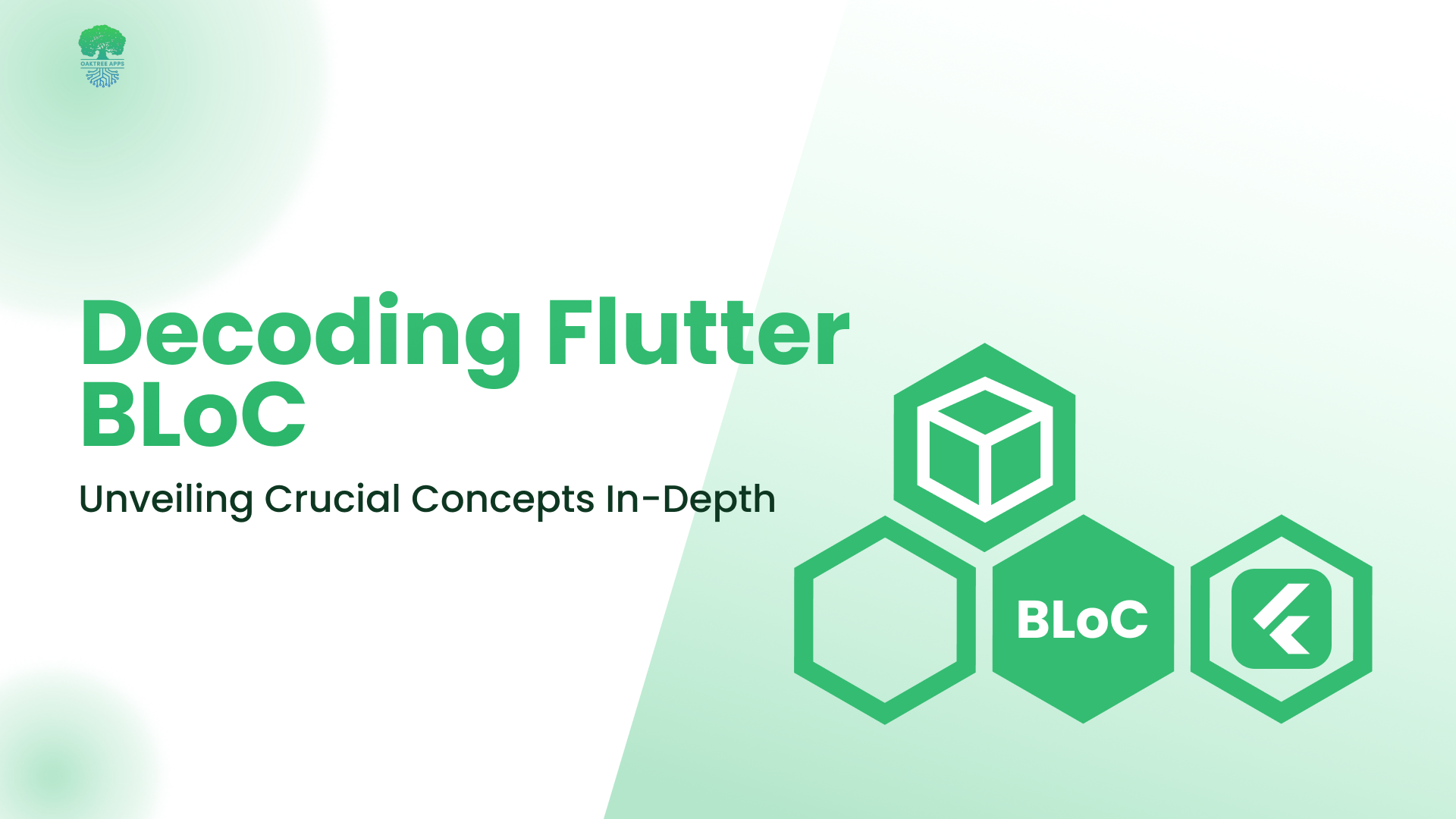
Introduction
Designing the structure of a cross-platform mobile app is a hotly debated topic in Flutter app development, with various architectural patterns in play. For iOS and Android developers, Model-View-Controller (MVC) has long been the default choice, where the model and view are separated, with the controller facilitating communication between them.
Flutter introduces a new reactive paradigm that doesn't align neatly with MVC. From the Flutter community emerges a variation of this classical pattern known as BLoC, short for Business Logic Components.
BLoC's core idea revolves around representing everything in the app as a stream of events: Widgets emit events, and other widgets respond to them, with BLoC orchestrating the conversation. What's particularly appealing is that Dart, the language behind Flutter, natively supports working with streams, eliminating the need for extra plugins or custom syntax.
Understanding BLoC in Flutter
Bloc is a design pattern that helps separate business logic from the presentation layer in Flutter applications. It allows developers to manage the application's state more efficiently by ensuring that there is a clear representation of the state for every interaction or scenario within the app. This can include displaying loading animations during data fetching or showing pop-ups for network connectivity issues.
In Flutter, a state management library called Bloc, created and maintained by Felix Angelo, is often used to implement the Bloc design pattern. It simplifies the process of managing the application's state and helps developers ensure a consistent and responsive user experience by separating the concerns of presentation and business logic.
Why Use Bloc
BLoC’s role extends far beyond nomenclature. BLoC acts as a bridge, connecting the intricate world of business logic with the user interface, all while keeping them neatly separated.
Have you ever experienced the frustration of launching an application that inexplicably crashes? These challenges can manifest as clicking a button and encountering a freeze lasting five to ten seconds, all without any informative feedback displayed in the user interface. Moreover, there might be instances where an application, designed to function seamlessly with an internet connection, falters when connectivity is absent.
If your answer to the above queries is affirmative, you're well-acquainted with the exasperation users feel when they're left without any visual cues or interaction within an application. The root issue often lies in code that lacks clarity, organization, maintainability, and testability. Frequently, app developers prioritize short-term development goals at the expense of long-term maintenance.
By applying BLoC we can ensure that the codebase remains resilient and adaptable over time.
Separation of Concerns: Bloc enforces a clear separation of concerns within your codebase. It cleanly divides the application into three distinct layers: the presentation layer (UI), the business logic layer, and the data layer. This separation enhances code readability and maintainability.
Reusability: Bloc facilitates the reusability of your code. Business logic encapsulated within Blocs can be reused across different parts of your application or even in entirely different projects, reducing redundant coding efforts.
Predictable State Management: Bloc provides a structured approach to state management. It ensures that your application's state transitions are explicit and predictable, which simplifies debugging and reduces the likelihood of unexpected crashes or behavior.
Testability: With Bloc, it's easier to write unit tests for your application's business logic. The separation of business logic from the user interface makes it simple to test individual components in isolation, ensuring the reliability of your code.
Scalability: As your application evolves and grows in complexity, Bloc scales with it. It accommodates additional features and changes in requirements without causing a ripple effect throughout your codebase.
Improved User Experience: Bloc enhances the user experience by allowing for responsive UI updates. It ensures that users receive visual feedback, such as loading indicators or error messages, during various app interactions.
Maintainability: By adhering to the Bloc architecture, your codebase becomes more maintainable in the long run. It's easier for developers to understand, update, and extend the application without introducing unnecessary complexity.
Supercharge your Flutter app development with BLoC architecture!
Elevate performance, streamline state management, and enhance scalability
Talk to Flutter ExpertsThe Significance of BLoC in Modern App Development
BLoC has quickly risen to prominence, and its significance cannot be overstated:
- Modular and Maintainable Code
- BLoC helps you break down your app's functionality into manageable units, making it easier to develop and maintain.
- With a well-structured BLoC, you can swiftly address issues, add new features, and extend your app's capabilities without causing a cascade of errors.
- Enhanced Code Reusability
- Imagine writing code once and deploying it across multiple platforms. BLoC empowers you to do just that, thanks to its platform-agnostic nature.
- The same BLoC can be utilized on iOS, Android, and the web, reducing development time and effort.
3. Responsive and Predictable UI
- BLoC ensures that your app's user interface remains responsive and predictable. Changes in state are managed efficiently, resulting in a seamless user experience.
- The separation of concerns offered by BLoC enhances your app's overall stability.
4. Streamlining Platform Migration
- For startup and mid-sized businesses contemplating a transition to Flutter, BLoC simplifies the process. It allows for the gradual integration of Flutter components, ensuring a smoother migration.
The Anatomy of a BLoC
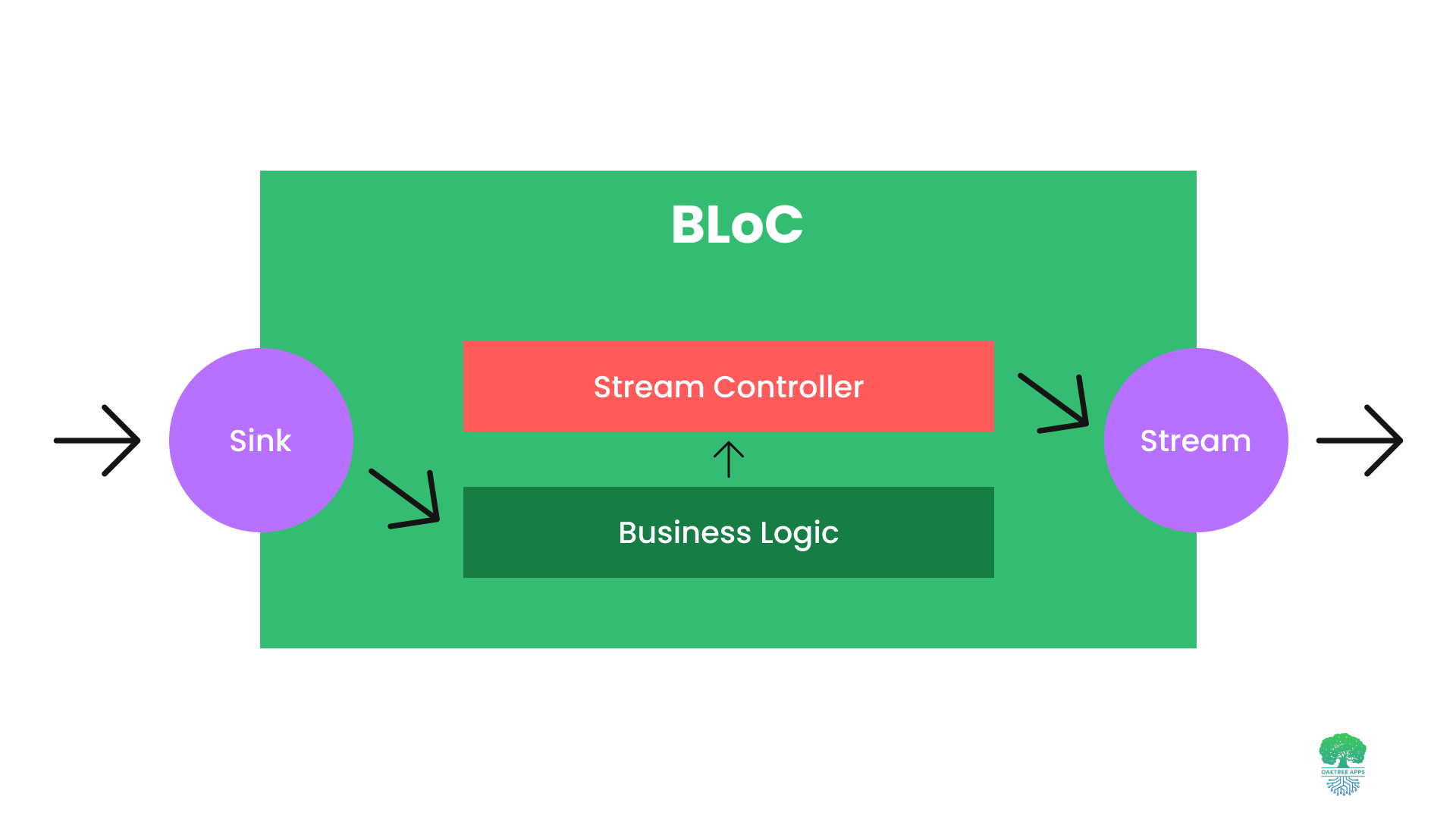
Let’s explore the essential components of a BLoC: the Sink, StreamController, and Stream. These elements are the building blocks of the BLoC pattern, orchestrating the flow of data within your Flutter applications.
1. Sink:
- Think of a Sink as the entry point for data into your BLoC. It's where you pour in information that needs to be processed by the business logic. You can imagine it as a faucet, letting data flow into your BLoC for further handling.
2. StreamController:
- The StreamController acts as the conductor of your data orchestra. It manages the flow of data from the Sink to the Stream and vice versa. This component plays a pivotal role in coordinating the movement of information within your BLoC.
3. Stream:
- Streams are like the channels through which data flows within your BLoC. They carry information from the StreamController to your UI components or any other parts of your app that need access to this data. Streams can be thought of as the pipes connecting the various parts of your app.
How Bloc Works
While streams and sinks are fundamental to Dart's asynchronous programming model, Bloc is a higher-level architectural pattern that leverages streams and sinks for state management. Bloc provides a structured way to handle application logic and state, making it easier to build and maintain complex Flutter applications. Let’s see how it works with an example:
Step 1: Triggering an Event
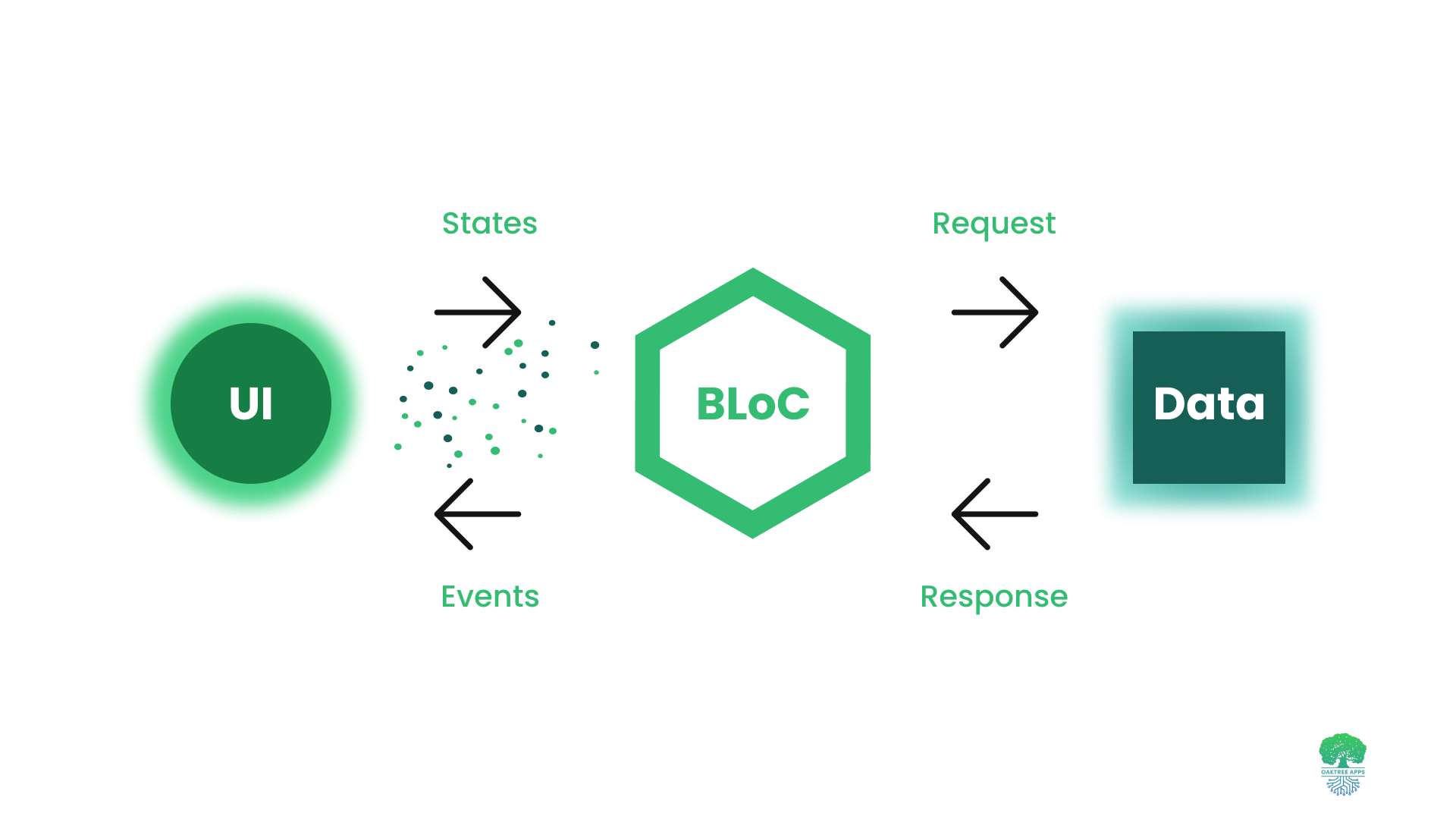
In your Flutter app, events are triggered when users interact with the user interface. For example, if you have a button to retrieve a list of books, an event is generated when the user clicks that button. Here's a simple Flutter code snippet to illustrate this:
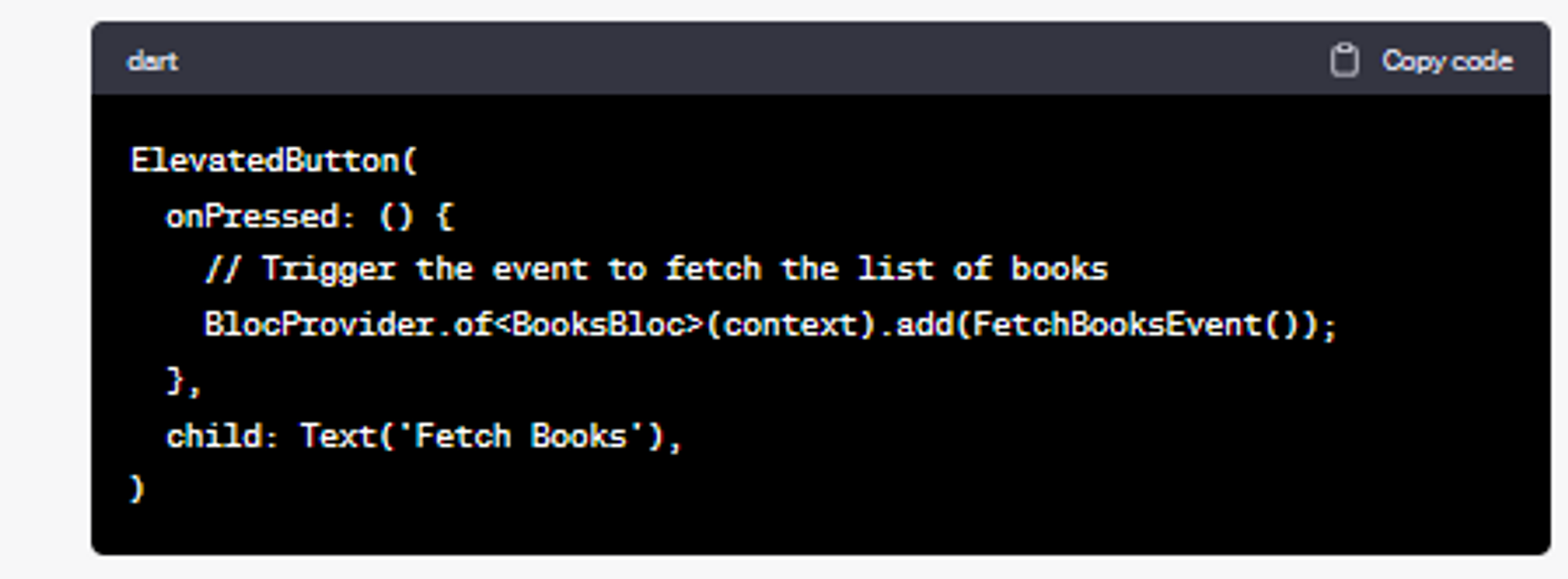
In this code, when the button is pressed, it triggers a FetchBooksEvent, which is then processed by the BooksBloc.
Step 2: Informing the Bloc
In the Flutter Bloc pattern, when you use the app, events are created to initiate interactions. For instance, if you want to fetch a list of books, you would generate an event when the user clicks a dedicated button.
Step 3: Data Retrieval
The Bloc takes charge of handling these events. It communicates with external sources like repositories to fetch the required data. Here's an example code snippet for this part:
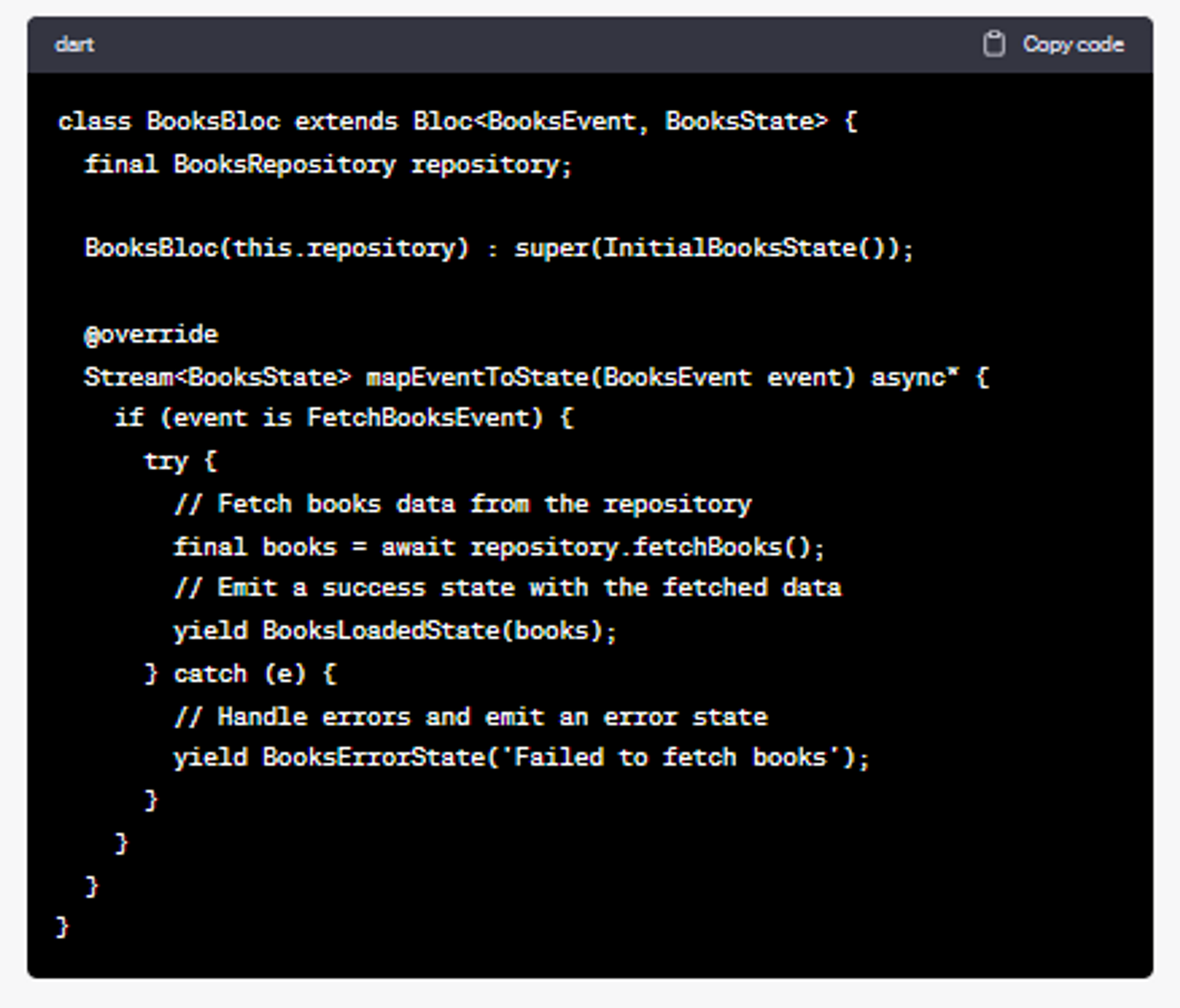
Here, the BooksBloc processes the FetchBooksEvent, retrieves data from the repository, and emits a state accordingly.
Step 4: Determining the State
In the Bloc, once an event is triggered, it's the Bloc's responsibility to request data, often from a repository. If successful, it emits a 'Success' state; otherwise, it emits an 'Error' state.
Step 5: Emitting a State
When the Bloc has the necessary data, it emits a corresponding state, such as 'Success' or 'Error,' based on the outcome of the data retrieval process.
Step 6: Reacting in the View
The view listens to these emitted states and reacts accordingly. Here's an example of how you can rebuild the UI based on different states:
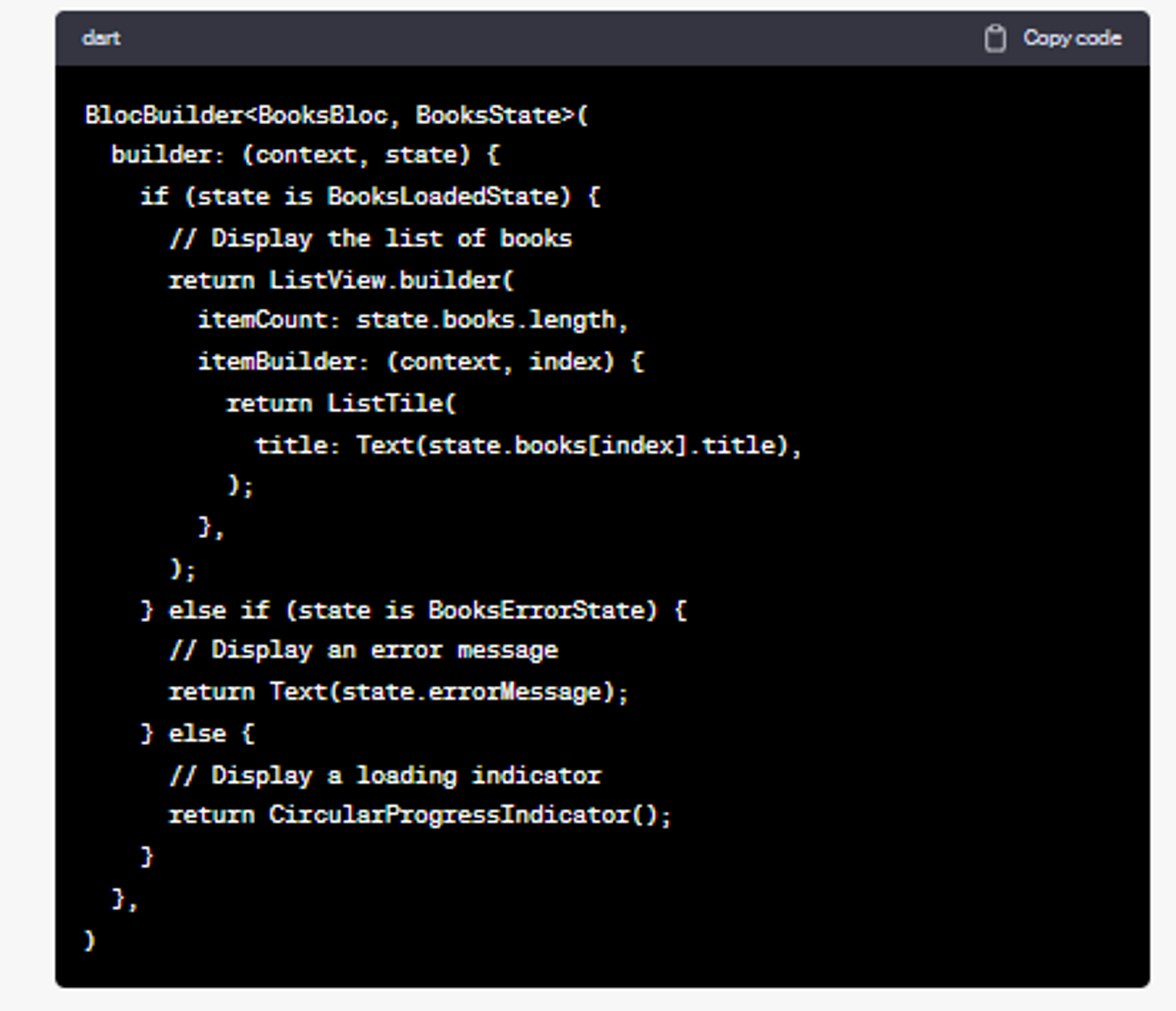
Here, the UI rebuilds differently based on the state emitted by the Bloc.
Now, let's tie it all together:
In the Flutter Bloc pattern, events are triggered by user interactions. For instance, you can create an event when a user clicks a button to retrieve a list of books. The BooksBloc then processes these events, requesting data, and emitting states like 'Success' or 'Error' based on the outcome. In the view, you can listen to these states and dynamically rebuild the UI to display the fetched data or show appropriate error messages or loading indicators.
BLoC vs. Other State Management Approaches
In the realm of state management for Flutter applications, BLoC is not the sole player on the stage. There are alternative approaches like Provider and Redux, each with its own set of strengths and weaknesses. Let's compare these options and guide you in choosing the right state management solution for your project.
BLoC: The Power of Separation
- Strengths:
- BLoC excels in maintaining a clear separation between business logic and UI components. This separation enhances code organization and maintainability.
- It's highly suitable for complex apps with numerous asynchronous operations, thanks to its reliance on Streams and Sinks.
- Weaknesses:
- BLoC can introduce some boilerplate code, making it appear more complex, especially for simple applications.
- While it provides robust state management, the learning curve for beginners might be steeper compared to other solutions.
Provider: Simplified State Management
- Strengths:
- Provider is known for its simplicity and ease of use. It's an excellent choice for smaller projects and developers new to state management.
- It eliminates some of the boilerplate code associated with BLoC, making it more accessible.
- Weaknesses:
- Provider's simplicity can become a limitation for larger and more complex applications, as it might not offer the same level of organization and structure as BLoC.
- Handling asynchronous operations can be more challenging with Provider compared to BLoC.
Redux: The Predictable State Container
- Strengths:
- Redux provides a highly predictable and centralized approach to state management, making it ideal for apps with complex and interconnected data flows.
- It enforces strict immutability, reducing the risk of unintended side effects.
- Weaknesses:
- Redux can introduce a significant amount of boilerplate code, which might be overwhelming for smaller projects.
- The learning curve for Redux, especially for developers new to the concept of state management, can be steep.
Real-World Use Cases and Success Stories
BLoC is not just a theoretical concept; it has been applied successfully in numerous real-world scenarios, delivering tangible benefis. Here are some examples:
1. E-commerce Apps:
- E-commerce apps often handle a complex array of user interactions, from product searches to cart management. BLoC helps streamline these processes and provides a seamless shopping experience.
2. Finance and Banking Apps:
- Security and real-time data updates are critical in financial apps. BLoC ensures data consistency and responsiveness, making it a valuable choice for this sector.
3. Social Networking Apps:
- Social networking apps require dynamic updates, notifications, and real-time messaging. BLoC's event-driven architecture suits these requirements perfectly.
4. News and Media Apps:
- News apps rely on frequent content updates and user preferences. BLoC helps manage content distribution and user interactions efficiently.
Conclusion
Effective state management is a fundamental aspect of developing Flutter applications, and Flutter Bloc emerges as a compelling choice. As we've delved into its key concepts in detail, it becomes evident that using Flutter Bloc need not be a complex endeavor. Understanding its core principles empowers you to harness its potential effectively.
Flutter Bloc offers a versatile toolkit for managing your app's views and widgets. Whether you prefer crafting small, specialized blocs for cleaner and more maintainable code or opt for larger blocs that handle multiple aspects of your app's logic, Flutter Bloc provides the flexibility to suit your specific needs.